Python Read a Text File Structured Like an Object
To read a text file in Python:
- Open the file.
- Read the lines from the file.
- Close the file.
For example, let'due south read a text file called example.txt from the same folder of the code file:
with open up('example.txt') as file: lines = file.readlines()
At present the lines variable stores all the lines from example.txt as a list of strings.
This is the quick answer. To larn more than details about reading files in Python, delight read along.
Reading Text Files in Python
Reading a text file into your Python plan follows this process:
- Open the file with the built-in open() function by specifying the path of the file into the call.
- Read the text from the file using one of these methods: read(), readline(), readlines().
- Shut the file using the shut() method. Yous can let Python handle closing the file automatically by opening the file using the with statement.
one. How to Utilize the open() Function in Python
The basic syntax for calling the open() function is:
open(path_to_a_file, mode)
Where:
- path_to_a_file is the path to the file yous want to open. For example Desktop/Python/example.txt. If your python plan file is in the same binder as the text file, the path is just the proper name of the file.
- manner specifies in which state you want to open up the file. There are multiple options. Merely as yous're interested in reading a file, y'all but need the mode 'r'.
The open() function returns an iterable file object with which you can hands read the contents of the file.
For instance, if you accept a file called example.txt in the same folder as your code file, yous can open it by:
file = open("example.txt", "r")
Now the file is opened, but not used in whatsoever useful fashion all the same.
Next, permit's take a look at how to actually read the opened file in Python.
ii. File Reading Methods in Python
To read an opened file, let'south focus on the 3 different text reading methods: read(), readline(), and readlines():
- read() reads all the text from a file into a single cord and returns the string.
- readline() reads the file line by line and returns each line as a separate string.
- readlines() reads the file line by line and returns the whole file as a listing of strings, where each string is a line from the file.
Later you lot are going to run into examples of each of these methods.
For case, let'southward read the contents of a file called "instance.txt" to a variable equally a string:
file = open("example.txt") contents = file.read()
Now the contents variable has any is inside the file as a single long string.
3. Always Close the File in Python
In Python, an opened file remains open as long as you lot don't close it. So brand sure to shut the file after using information technology. This can be done with the shut() method. This is important because the program can crash or the file can decadent if left hanging open up.
file.close()
Y'all can besides let Python take intendance of closing the file by using the with statement when dealing with files. In this instance, you don't need the close() method at all.
The with statement construction looks like this:
with open(path_to_file) equally file: #read the file hither
Using the with statement is so convenient and conventional, that nosotros are going to stick with it for the rest of the guide.
Now you empathise the basics of reading files in Python. Next, let'southward have a look at reading files in action using the dissimilar reading functions.
Using the File Reading Methods in Python
To repeat the following examples, create a folder that has the following two files:
- A reader.py file for reading text files.
- An example.txt file from where your program reads the text.
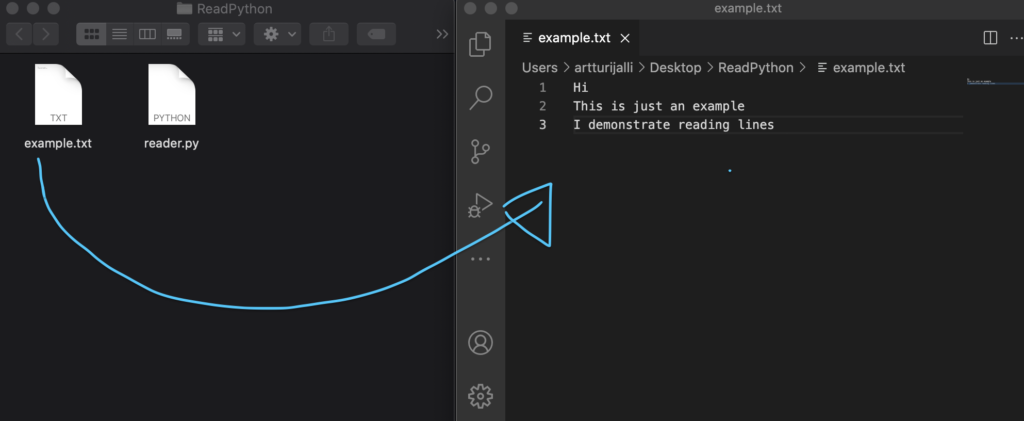
Also, write some text on multiple lines into the example.txt file.
The read() method in Python
To read a file to a unmarried string, use the read() method. As discussed higher up, this reads whatever is in the file as a single long string into your program.
For example, let's read and print out the contents of the example.txt file:
with open("example.txt") as file: contents = file.read() impress(contents)
Running this slice of code displays the contents of case.txt in the console:
Hello This is only an case I demonstrate reading lines
The readline() Method in Python
To read a file ane line at a time, utilize the readline() method. This reads the current line in the opened file and moves the line pointer to the next line. To read the whole file, employ a while loop to read each line and motion the file pointer until the end of the file is reached.
For instance:
with open up('example.txt') as file: next_line = file.readline() while next_line: print(next_line) next_line = file.readline()
As a result, this program prints out the lines one by ane as the while loop gain:
Hello This is just an example I'thou demonstrate reading lines
The readlines() Method in Python
To read all the lines of a file into a listing of strings, utilize the readlines() method.
When y'all have read the lines, you tin can loop through the list of lines and print them out for example:
with open('instance.txt') as file: lines = file.readlines() line_num = 0 for line in lines: line_num += 1 print(f"line {line_num}: {line}")
This displays each line in the panel:
line 1: Hello line 2: This is merely an example line 3: I'm demonstrate reading lines
Use a For Loop to Read an Opened File
You just learned about 3 different methods you can utilize to read a file into your Python program.
It is good to realize that the open() role returns an iterable object. This means that you can loop through an opened file just like you would loop through a list in Python.
For example:
with open('example.txt') as file: for line in file: print(line)
Output:
Hi This is just an example I'm demonstrate reading lines
As y'all tin can see, yous did not need to use any of the born file reading methods. Instead, you used a for loop to run through the file line by line.
Decision
Today y'all learned how to read a text file into your Python program.
To epitomize, reading files follows these three steps:
- Use theopen() function with the'r' style to open a text file.
- Use one of these three methods:read(),readline(), orreadlines() to read the file.
- Close the file after reading it using theclose() method or let Python practice information technology automatically by using thewith statement.
Conventionally, you tin besides use the with argument to reading a file. This automatically closes the file for y'all and then you do not need to worry nearly the 3rd step.
Cheers for reading. Happy coding!
Farther Reading
Python—How to Write to a File
10 Useful Python Snippets to Code Like a Pro
harperaptaidene68.blogspot.com
Source: https://www.codingem.com/read-textfile-into-python-program/